Fonts & typography#
Font stack#
Text & Headings#
For text & headings, Bumbag uses the operating system's sans serif fonts.
font-family: system-ui, -apple-system, BlinkMacSystemFont, 'Segoe UI', Roboto, Oxygen, Ubuntu, Cantarell, 'Fira Sans', 'Droid Sans', 'Helvetica Neue', sans-serif;
Here is how they look on an per-OS basis:
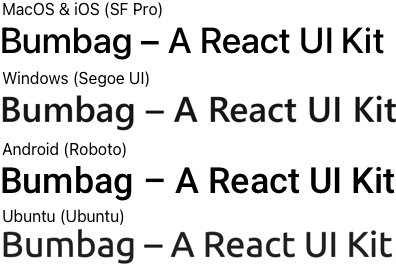
Mono (code blocks)#
For code blocks, Bumbag uses the operating system's mono fonts.
font-family: 'SF Mono', 'Segoe UI Mono', 'Roboto Mono', Menlo, Courier, monospace;
Here is how they look on an per-OS basis:
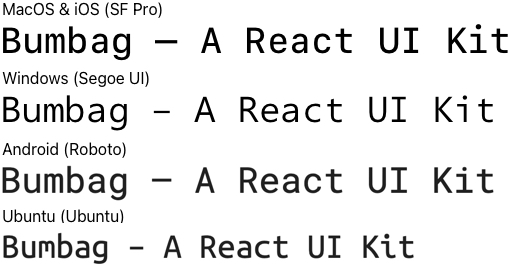
Modifying the theme fonts#
You can change the font families through the Bumbag theme:
import { css, Provider } from 'bumbag';const theme = {fonts: {default: "system-ui, sans-serif",heading: "'Comic Sans MS', sans-serif",mono: "Menlo, Courier, monospace",}}export default () => (<Provider theme={theme}>{/* your app... */}</Provider>)
Note: If you are changing the theme fonts, you may want to also apply their font metrics
External fonts#
Bumbag supports importing third-party fonts (e.g. Google Fonts). Click here to see a list of Google Fonts.
You can do this via the fonts.importUrls
attribute:
import { css, Provider } from 'bumbag';const theme = {fonts: {importUrls: ['https://fonts.googleapis.com/css2?family=Lato:wght@400;700;900&display=swap'],default: "'Lato', system-ui, sans-serif"}}export default () => (<Provider theme={theme}>{/* your app... */}</Provider>)
Note: If you are changing the theme fonts, you may want to also apply their font metrics
Font trimming & metrics#
Thanks to Capsize, Bumbag trims the unpredictable space above capital letters and below the font baseline.
Bumbag provides font metrics out of the box for the default theme fonts. However, if you modify the fonts in the theme, you will need to provide font metrics for your custom font. If you don't provide font metrics, your custom fonts will still work but you may see inconsistencies in the white space trimming of the text.
To obtain font metrics for your custom font, follow these steps:
- Head to the Capsize website
- Choose or upload your font
- Ignore the Adjust size & spacing section, and just head to Apply the styles
- Under Apply the styles, you will see a
fontMetrics
variable - Use the
fontMetrics
value in the Bumbag theme!
Below is a full example of applying the font metrics to the theme with custom fonts:
import { css, Provider } from 'bumbag';const theme = {fonts: {importUrls: ['https://fonts.googleapis.com/css2?family=Lato:wght@400;700;900&display=swap','https://fonts.googleapis.com/css2?family=Merriweather:wght@700&display=swap'],default: "Lato, sans-serif",heading: "Merriweather, sans"},fontMetrics: {default: {capHeight: 1433,ascent: 1974,descent: -426,lineGap: 0,unitsPerEm: 2000},heading: {capHeight: 743,ascent: 984,descent: -273,lineGap: 0,unitsPerEm: 1000}}}export default () => (<Provider theme={theme}>{/* your app... */}</Provider>)
Font families#
Accessing font families#
You can access the font families through themeable components:
With applyTheme
#
import { Text, applyTheme } from 'bumbag';const MonoText = applyTheme(Box, {styles: {base: {fontFamily: 'mono'}}})<MonoText>I have the mono font family</MonoText>
With styled
#
import { Text, styled, fontFamily } from 'bumbag';const LargeText = styled(Text.Block)`font-family: ${fontFamily('mono')};`;<LargeText>I am bold</LargeText>
Using style props#
or, you can use style props:
Font weights#
Bumbag currently has three preset font weights: normal
, semibold
and bold
.
You can also specify a font weight in the range of 100
-900
.
You can also add your own custom font weights in the theme config if you wish.
Accessing font weights#
You can access the font weights through themeable components:
With applyTheme
#
import { Text, applyTheme } from 'bumbag';const BoldText = applyTheme(Text.Block, {styles: {base: {fontWeight: 'bold'}}});<BoldText>I am bold</BoldText>
With styled
#
import { Text, styled, fontWeight } from 'bumbag';const LargeText = styled(Text.Block)`font-size: ${fontWeight('bold')};`;<LargeText>I am bold</LargeText>
Using style props#
or, you can use style props:
Font sizes#
Bumbag uses a scale from 100
-900
for font sizes. We use a numeric scale, instead of a contextual scale (like small
, medium
, etc) to maximize flexibility so you can easily add sizes inbetween if you need.
200
(16px) is the base font size.
Accessing font sizes#
You can access the font sizes through themeable components:
With applyTheme
#
import { Text, applyTheme } from 'bumbag';const LargeText = applyTheme(Text, {styles: {base: {fontSize: '400'}}});<LargeText>I am bold</LargeText>
With styled
#
import { Text, styled, fontSize } from 'bumbag';const LargeText = styled(Text)`font-size: ${fontSize('400')};`;<LargeText>I am bold</LargeText>
Using style props#
or, you can use style props:
Line heights#
A numerical scale (from none
to 600
) is also used for line heights.
Accessing line heights#
You can access the line heights through themeable components:
With applyTheme
#
import { Text, applyTheme } from 'bumbag';const CustomText = applyTheme(Text, {styles: {base: {lineHeight: 'none'}}});<CustomText>Hello<br/>World</CustomText>
With styled
#
import { Text, styled, lineHeight } from 'bumbag';const CustomText = styled(Text)`font-size: ${lineHeight('none')};`;<CustomText>Hello<br/>World</CustomText>
Using style props#
or, you can use style props:
World
Letter spacings#
A numerical scale (from 100
to 700
) is also used for letter spacings.
Accessing letter spacings#
You can access the letter spacings through themeable components:
With applyTheme
#
import { Text, applyTheme } from 'bumbag';const CustomText = applyTheme(Text, {styles: {base: {letterSpacing: '100'}}});<CustomText>Hello World</CustomText>
With styled
#
import { Text, styled, letterSpacing } from 'bumbag';const CustomText = styled(Text)`font-size: ${letterSpacing('100')};`;<CustomText>Hello World</CustomText>
Using style props#
or, you can use style props:
Theming#
Schema#
const theme = {fonts: {importUrls: Array<string>,default: string,heading: string,mono: string},fontMetrics: {[font: string]: {capHeight: number,ascent: number,descent: number,lineGap: number,unitsPerEm: number}},fontWeights: {normal: number,semibold: number,bold: number,[key: string]: number},fontSizes: {100: number,150: number,200: number,300: number,400: number,500: number,600: number,700: number,800: number,900: number,[key: string]: number},lineHeights: {none: number,default: number,100: number,200: number,300: number,400: number,500: number,600: number,[key: string]: number},letterSpacings: {default: string,100: string,200: string,300: string,400: string,500: string,600: string,[key: string]: string}}
Example#
const theme = {fonts: {importUrls: ['https://fonts.googleapis.com/css2?family=Lato:wght@400;700;900&display=swap','https://fonts.googleapis.com/css2?family=Merriweather:wght@700&display=swap'],default: "'Lato', sans-serif",heading: "'Merriweather', sans-serif",mono: "Menlo, Courier, monospace",},fontMetrics: {default: {capHeight: 1433,ascent: 1974,descent: -426,lineGap: 0,unitsPerEm: 2000},heading: {capHeight: 743,ascent: 984,descent: -273,lineGap: 0,unitsPerEm: 1000}},fontWeights: {normal: 300,semibold: 400,bold: 500,bolder: 600},fontSizes: {50: 0.5,950: 5,extraSmall: 0.25},lineHeights: {700: 3,extraSmall: 0.8},letterSpacings: {800: '0.2em',extraSmall: '-0.075em'},}