Fonts & typography#
Font stack#
Bumbag Native uses the operating system's sans serif fonts as it's default font. This will be different for each OS.
Adding custom fonts#
To add a custom font to Bumbag Native, you will need to do the following:
- Add the font to the React Native project
- Add the font to the web project (optional)
- Add the font to the theme
1. Adding fonts to React Native#
Add the assets into your project#
In order to add a custom font to React Native, you will need to first place your font assets (.ttf, .otf, or whatever) into your src/assets
folder.
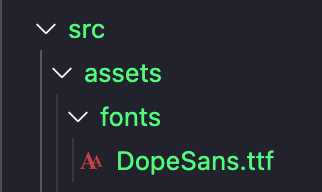
Add/update your React Native config#
You will then need to update your React Native config file to support the fonts folder.
Add the following to the react-native.config.js
file:
module.exports = {assets: ['./src/assets/fonts'],};
Link assets to the project#
After that, you will need to link the assets to your project:
npx react-native link
2. Adding fonts to web#
TODO
3. Adding fonts to the theme#
You can change the font families through the Bumbag Native theme:
import { Provider } from 'bumbag-native';const theme = {fonts: {default: 'DopeSans',heading: 'DopeSansSerif'}}export default () => (<Provider theme={theme}>{/* your app... */}</Provider>)
Font families#
Accessing font families#
With style props#
With applyTheme
#
Font weights#
Bumbag currently has three preset font weights: normal
, semibold
and bold
.
You can also specify a font weight in the range of 100
-900
.
You can also add your own custom font weights in the theme config if you wish.
Custom font weights#
If you have set up a custom font with your app, and want to have the ability to specify a range of weights for that font, you will need to configure the font weights to do so.
This can be configured via the theme.fontWeights
property in the theme.
The fontWeights
theme attributes evaluates to a font
(key) / fontWeights
(value) pair.
An example is seen below:
import { Provider } from 'bumbag-native';const theme = {fonts: {default: 'DopeSans',heading: 'DopeSansSerif'},fontWeights: {default: {normal: {fontFamilySuffix: 'Regular' // `<Text fontWeight="normal">` styles evaluates to `fontFamily: DopeSans-Regular`},semibold: {fontFamilySuffix: 'SemiBold' // `<Text fontWeight="semibold">` styles evaluates to `fontFamily: DopeSans-SemiBold`},bold: {fontFamilySuffix: 'Bold' // `<Text fontWeight="bold">` styles evaluates to `fontFamily: DopeSans-Bold`}},heading: {normal: {fontFamilySuffix: 'Regular' // `<Text font="heading" fontWeight="normal">` styles evaluates to `fontFamily: DopeSansSerif-Regular`},semibold: {fontFamilySuffix: 'Regular' // `<Text font="heading" fontWeight="semibold">` styles evaluates to `fontFamily: DopeSansSerif-SemiBold`},bold: {fontFamilySuffix: 'Regular' // `<Text font="heading" fontWeight="bold">` styles evaluates to `fontFamily: DopeSansSerif-Bold`},}}}export default () => (<Provider theme={theme}>{/* your app... */}</Provider>)
Accessing font weights#
With style props#
With applyTheme
#
Font sizes#
Bumbag uses a scale from 100
-900
for font sizes. We use a numeric scale, instead of a contextual scale (like small
, medium
, etc) to maximize flexibility so you can easily add sizes inbetween if you need.
200
(16px) is the base font size.
Accessing font sizes#
With style props#
With applyTheme
#
import { Text, applyTheme } from 'bumbag';const LargeText = applyTheme(Text, {styles: {base: {fontSize: '400'}}});<LargeText>I am bold</LargeText>
Theming#
Schema#
const theme = {fonts: {default: string,heading: string,mono: string},fontWeights: {[font: string]: {normal: {fontFamilySuffix: string,fontWeight: string},semibold: {fontFamilySuffix: string,fontWeight: string},bold: {fontFamilySuffix: string,fontWeight: string},[key: string]: {fontFamilySuffix: string,fontWeight: string}}},fontSizes: {100: number,150: number,200: number,300: number,400: number,500: number,600: number,700: number,800: number,900: number,[key: string]: number}}
Example#
const theme = {fonts: {default: "Lato",heading: "Merriweather"},fontWeights: {normal: 300,semibold: 400,bold: 500,bolder: 600},fontSizes: {50: 0.5,950: 5,extraSmall: 0.25}}