BottomSheet#
The BottomSheet
and it's respective components are exports of @gorhom/react-native-bottom-sheet
.
You can read more on the internal BottomSheet
functionalities here. As Bumbag inherits @gorhom/react-native-bottom-sheet
, it shares the same props, methods and API.
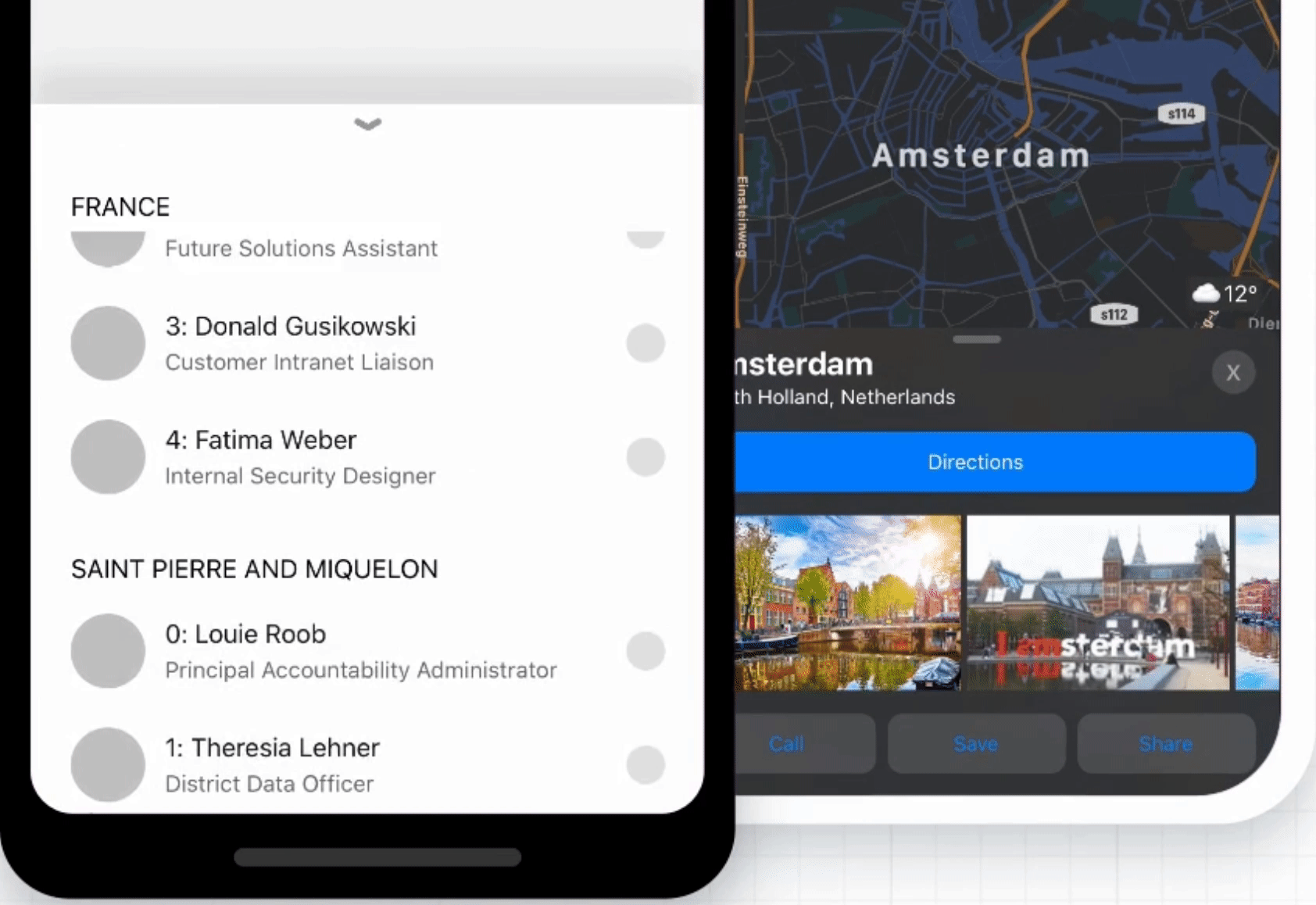
Compatibility#
iOS | Android | Web |
---|---|---|
✅ | ✅ | ❌ |
Install#
This component requires you to install
@bumbag-native/bottom-sheet
,react-native-gesture-handler
&react-native-reanimated
as a separate dependency.
npm install @bumbag-native/bottom-sheet react-native-reanimated react-native-gesture-handler
Ensure you have set up react-native-reanimated and react-native-gesture-handler in your project.
Import#
import { BottomSheet } from '@bumbag-native/bottom-sheet';
Usage#
A BottomSheet
in it's simplest form will render a sheet from the bottom with it's respective content.
<BottomSheet>Hello world</BottomSheet>
Snap points#
Points for the bottom sheet to snap to, points should be sorted from bottom to top. It accepts array of number, string or mix.
<BottomSheet snapPoints={['25%', '50%']}>Hello world</BottomSheet>
Methods#
snapTo#
Animate the sheet to one of the provided point from snapPoints
.
function Example() {const bottomSheetRef = React.useRef();return (<>{/* This will snap to 50% */}<Button onPress={() => bottomSheetRef.current.snapTo(1)}>Snap</Button><BottomSheet ref={bottomSheetRef} snapPoints={['25%', '50%']}>Hello world</BottomSheet></>)}
expand#
Animate the sheet to the maximum provided point from snapPoints
.
function Example() {const bottomSheetRef = React.useRef();return (<>{/* This will expand to 50% */}<Button onPress={() => bottomSheetRef.current.expand()}>Expand</Button><BottomSheet ref={bottomSheetRef} snapPoints={['25%', '50%']}>Hello world</BottomSheet></>)}
collapse#
Animate the sheet to the minimum provided point from snapPoints
.
function Example() {const bottomSheetRef = React.useRef();return (<>{/* This will collapse to 25% */}<Button onPress={() => bottomSheetRef.current.expand()}>Expand</Button><BottomSheet ref={bottomSheetRef} snapPoints={['25%', '50%']}>Hello world</BottomSheet></>)}
close#
Close the sheet.
function Example() {const bottomSheetRef = React.useRef();return (<><Button onPress={() => bottomSheetRef.current.close()}>Close</Button><BottomSheet ref={bottomSheetRef} snapPoints={['25%', '50%']}>Hello world</BottomSheet></>)}
Hooks#
useBottomSheet#
This hook provides all the bottom sheet public methods, to the internal sheet content or handle.
This hook works at any component inside the
BottomSheet
.
import { useBottomSheet } from '@bumbag-native/bottom-sheet';function Example() {const { expand } = useBottomSheet();return (<Box><Button onPress={expand}>Expand bottom sheet</Button></Box>)}
useBottomSheetSpringConfigs#
Generate animation spring configs.
import { BottomSheet, useBottomSheetSpringConfigs } from '@bumbag-native/bottom-sheet';function Example() {const animationConfigs = useBottomSheetSpringConfigs({damping: 80,overshootClamping: true,restDisplacementThreshold: 0.1,restSpeedThreshold: 0.1,stiffness: 500,});return (<BottomSheetanimationConfigs={animationConfigs}>Hello world</BottomSheet>)}
useBottomSheetTimingConfigs#
Generate animation timing configs.
import BottomSheet, { useBottomSheetTimingConfigs } from '@bumbag-native/bottom-sheet';import { Easing } from 'react-native-reanimated';function Example() {const animationConfigs = useBottomSheetTimingConfigs({duration: 250,easing: Easing.exp,});return (<BottomSheetanimationConfigs={animationConfigs}>Hello world</BottomSheet>)}
Custom handle#
You can customise the handle via the renderHandle
prop.
<BottomSheetrenderHandle={() =><Box backgroundColor="gray" borderRadius="2px" height="major-1" width="major-5" />}>Hello world</BottomSheet>