BottomSheet.Modal#
The BottomSheet.Modal
and it's respective components are exports of @gorhom/react-native-bottom-sheet
.
You can read more on the internal BottomSheet.Modal
functionalities here. As Bumbag inherits @gorhom/react-native-bottom-sheet
, it shares the same props, methods and API.
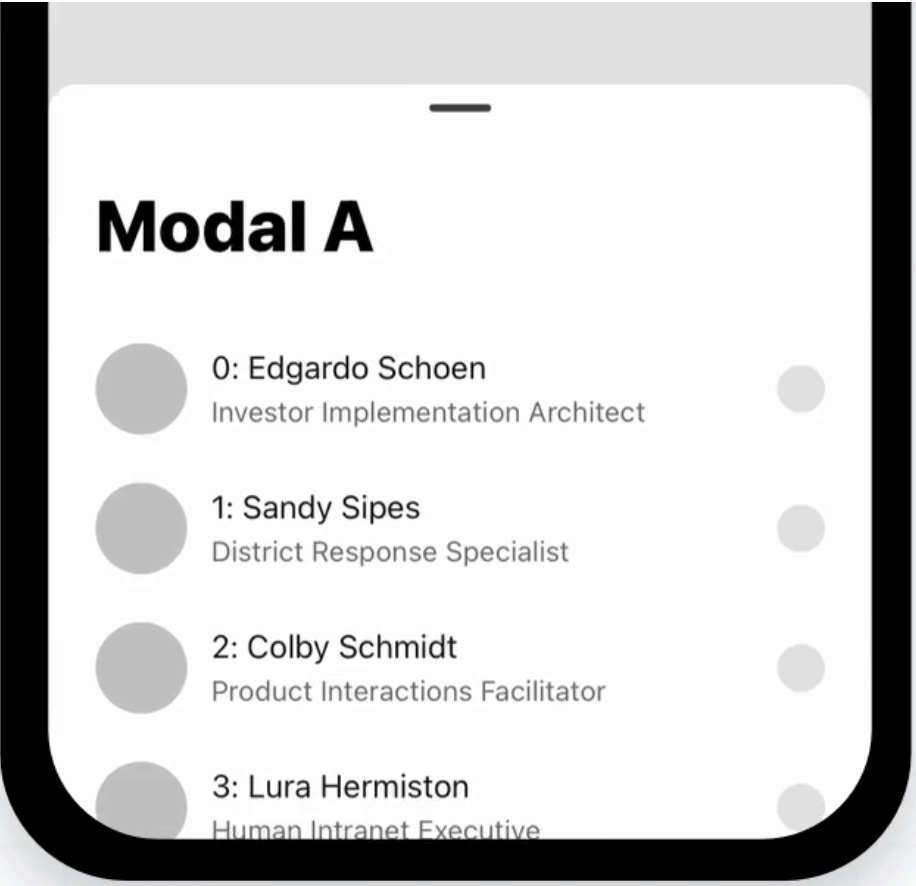
Compatibility#
iOS | Android | Web |
---|---|---|
✅ | ✅ | ❌ |
Install#
This component requires you to install
@bumbag-native/bottom-sheet
,react-native-gesture-handler
&react-native-reanimated
as a separate dependency.
npm install @bumbag-native/bottom-sheet react-native-reanimated react-native-gesture-handler
Ensure you have set up react-native-reanimated and react-native-gesture-handler in your project.
Import#
import { BottomSheet } from '@bumbag-native/bottom-sheet';
Usage#
You can render a bottom sheet as a modal. It provides all of the BottomSheet
functionalities, with extra modal presentation functionalities.
To get started using bottom sheet modal's in your app, you will need to wrap your app in a BottomSheetModalProvider
:
import { BottomSheetModalProvider } from '@bumbag-native/bottom-sheet';function App() {return (<BottomSheetModalProvider>// ... your app</BottomSheetModalProvider>);}
Then you can start using BottomSheet.Modal
in your app:
import React from 'react';import { Button } from 'bumbag-native';import { BottomSheet } from '@bumbag-native/bottom-sheet';function Example() {const modalRef = React.useRef();return (<><Button onPress={() => modalRef.current.present()}>Show modal</Button><BottomSheet.Modal ref={modalRef}>Hello world!</BottomSheet.Modal></>)}
Methods#
present#
Mount and present the modal.
function Example() {const bottomSheetRef = React.useRef();return (<><Button onPress={() => bottomSheetRef.current.present()}>Present</Button><BottomSheet.Modal ref={bottomSheetRef}>Hello world</BottomSheet.Modal></>)}
dismiss#
Close and unmount the modal.
function Example() {const bottomSheetRef = React.useRef();return (<><Button onPress={() => bottomSheetRef.current.dismiss()}>Dismiss</Button><BottomSheet.Modal ref={bottomSheetRef}>Hello world</BottomSheet.Modal></>)}
Custom handles#
<BottomSheet.ModalrenderHandle={() =><Box backgroundColor="gray" borderRadius="2px" height="major-1" width="major-5" />}>Hello world</BottomSheet.Modal>